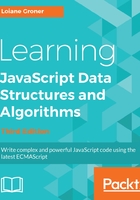
Array entries, keys, and values
ES2015 also introduced three ways of retrieving iterators from an array. The first one you will learn is the entries method.
The entries method returns @@iterator, which contains key/value pairs. The following is an example of how we can use this method:
let aEntries = numbers.entries(); // retrieve iterator of key/value console.log(aEntries.next().value); // [0, 1] - position 0, value 1 console.log(aEntries.next().value); // [1, 2] - position 1, value 2 console.log(aEntries.next().value); // [2, 3] - position 2, value 3
As the number array only contains numbers, key will be the position of the array, and value will be the value stored in the array index.
We can also use the following code as an alternative to the preceding code:
aEntries = numbers.entries(); for (const n of aEntries) { console.log(n); }
To be able to retrieve key/value pairs is very useful when we are working with sets, dictionaries, and hash maps. This functionality will be very useful to us in the later chapters of this book.
The keys method returns @@iterator, which contains the keys of the array. The following is an example of how we can use this method:
const aKeys = numbers.keys(); // retrieve iterator of keys console.log(aKeys.next()); // {value: 0, done: false } console.log(aKeys.next()); // {value: 1, done: false } console.log(aKeys.next()); // {value: 2, done: false }
For the numbers array, the keys will be the indexes of the array. Once there are no values to be iterated, the code aKeys.next() will return undefined as value and done as true. When done has the value false, it means that there are still more keys of the array to be iterated.
The values method returns @@iterator, which contains the values of the array. The following is an example of how we can use this method:
const aValues = numbers.values(); console.log(aValues.next()); // {value: 1, done: false } console.log(aValues.next()); // {value: 2, done: false } console.log(aValues.next()); // {value: 3, done: false }