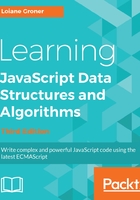
Running ES2015 modules in the browser and with Node.js
Let's try to run the 17-ES2015-ES6-Modules.js file with Node.js by changing the directory and then executing the node command as follows:
cd path-source-bundle/examples/chapter01 node 17-ES2015-ES6-Modules
We will get an error, SyntaxError: Unexpected token import. This is because at the time of writing this book, Node.js does not support ES2015 native modules. Node.js uses CommonJS module require syntax, and this means we need to transpile our ES2015 so Node can understand it. There are different tools we can use for this task. To keep things simple, we will use Babel CLI.
The best approach would be to create a local project and configure it to use Babel. Unfortunately, all of these details are not in the scope of this book (this is a subject for a Babel book). For our example, and to keep things simple, we will use Babel CLI globally by installing it using npm:
npm install -g babel-cli
If you use Linux or Mac OS, you might want to use sudo in the front of the command for admin access (sudo npm install -g babel-cli).
From inside the chapter01 directory, we will compile the three JavaScript files with modules we created previously to CommonJS transpile code with Babel, so we can use the code using Node.JS. We will transpile the file to the chapter01/lib folder using the following commands:
babel 17-CalcArea.js --out-dir lib babel 17-Book.js --out-dir lib babel 17-ES2015-ES6-Modules.js --out-dir lib
Next, let's create a new JavaScript file named 17-ES2015-ES6-Modules-node.js so we can use the area functions and the Book class:
const area = require('./lib/17-CalcArea'); const Book = require('./lib/17-Book'); console.log(area.circle(2)); console.log(area.square(2)); const myBook = new Book('some title'); myBook.printTitle();
The code is basically the same, but the difference is that since Node.js does not support the import syntax (for now), we need to use the require keyword.
To execute the code, we can use the following command:
node 17-ES2015-ES6-Modules-node
In the following screenshot, we can see the commands and the output, so we can confirm that the code works with Node.js:
