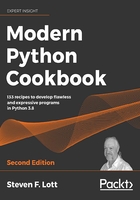
4 Built-In Data Structures Part 1: Lists and Sets
Python has a rich collection of built-in data structures. These data structures are sometimes called "containers" or "collections" because they contain a collection of inpidual items. These structures cover a wide variety of common programming situations.
We'll look at an overview of the various collections that are built-in and what problems they solve. After the overview, we will look at the list and set collections in detail in this chapter, and then dictionaries in Chapter 5, Built-In Data Structures Part 2: Dictionaries.
The built-in tuple and string types have been treated separately. These are sequences, making them similar in many ways to the list collection. In Chapter 1, Numbers, Strings, and Tuples, we emphasized the way strings and tuples behave more like immutable numbers than like the mutable list collection.
The next chapter will look at dictionaries, as well as some more advanced topics also related to lists and sets. In particular, it will look at how Python handles references to mutable collection objects. This has consequences in the way functions need to be defined that accept lists or sets as parameters.
In this chapter, we'll look at the following recipes, all related to Python's built-in data structures:
- Choosing a data structure
- Building lists – literals, appending, and comprehensions
- Slicing and dicing a list
- Deleting from a list – deleting, removing, popping, and filtering
- Writing list-related type hints
- Reversing a copy of a list
- Building sets – literals, adding, comprehensions, and operators
- Removing items from a set – remove(), pop(), and difference
- Writing set-related type hints