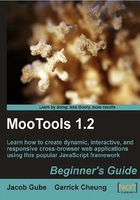
Using MooTools classes
Now that you know how to create a class in MooTools, it'll be a piece of cake to use the already-existing classes in the MooTools framework. You've already used a MooTools class, whether you know it or not, with the Options
class that we implemented in the Dogs
class.
Let's look at another class, the Chain
class, so that we can explore how to use MooTools classes. And we're going to hit three birds with one stone here: by covering the Chain
class to see how MooTools classes work in general, you'll also discover a key concept in the MooTools framework—chainability, and be able to witness the power of the Chain
class. Once we're done, it'll be a cinch to use other MooTools classes such as Fx
class for animation effects (we'll cover in greater detail in a later chapter).
The concept of chainability
Alright, so you caught me, chainability isn't a real word. Chainability refers to MooTools' ability to chain functions in sequence. It follows the concept of a Stack, a data structure that follows the principle of "last in, first out". Chaining allows you to create a stack of functions that you can execute in sequential order.
The Chain class
The Chain
class, as you would expect, is a class for dealing with a chain of functions. It contains the following useful methods:
A Chain example
Let's set up an example for showcasing the concept of chaining and the abilities of the Chain
class. We're going to create a box using a<div>
element that has a width and height of 50px and a background color of blue (hexadecimal value of #00f). For convenience, we're going to break my rule of separating style and structure that I discussed in the beginning of this chapter by declaring an inline style attribute. Here's our HTML markup for the<div>
element:
<div id="box" style="background-color: #00f; height:50px; width:50px;"> </div>
Note
Avoid using inline styles. It's a bad practice, and though development best practices for style and content separation is beyond the scope of this book, I'll make all the efforts I can in advocating the use of best practices. We'll break this rule in this case so that we're focused on the topic at hand and not our CSS and HTML.
Now that we have a nice<div>
box to work with, what are we going to do with it?
A look ahead: Chaining Fx .Tween
I don't think I can let you read any more chapters without seeing some cool animation effects. So I'm going to cheat a bit (I hope the editors of this book don't notice that I snuck in some animation effects in this chapter), and jump ahead to use a Fx.Tween
class, except that it's just like any other class in MooTools and that it deals with taking two CSS property values and transitions between them.
This is what we're going to do:
- Move the blue box to the right by tweening its left margin.
- Double its width (100px).
- Fade it out.
- Fade it back in.
- Tween it back to its original position.
We're going to do this by chaining these four methods one after the other.
Let's write out the methods now:
.start('margin-left', 150) .start('width', 100) .start(opacity, 0) .start(opacity, 1) .start('margin-left', 0)
Alright, so we have our HTML markup set up and we already know what methods we're going to stack. Let's get your chain on!