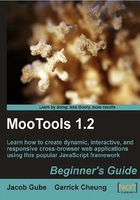
Creating MooTools classes
I've mentioned it, like, a million times already, but here I go again, MooTools is an object-oriented web application framework. And what's OOP without classes? Not OOP, that's for sure! MooTools is, to me, the only JavaScript library that effectively implements and promotes the use of classes.
What the heck is a class?
A class, in terms of object-oriented programming, is a definition of an object, or an object's blueprint, its design, its structure, its template, I can go on, but I think you get the picture. Classes are best described in real-world terms.
Real-world analogy
Let's say our class is "Dog". A dog can have a lot of traits, such as its name, its type (for example, Golden Retriever or Poodle), its age, and so on. In MooTools, these traits are called options, which is an object that contains default key/value pairs specific to a particular class.
A dog can also do a lot of things, such as bark, sit, or eat. In the context of JavaScript and classes, these are called class methods. A method is a function for a particular class or object. For example, myPoodle.bark()
can mean that we make my poodle bark by triggering the bark()
method.
Let's create a Dog
class in MooTools.
Creating a MooTools class
We create a MooTools class using the following format:
var ClassName = new Class({ properties });
So for our Dog
class, here's what we'll start with:
var Dog = new Class( { // Implements is a class property // upon which other classes methods will be added Implements : [ Options ], // Default options for our Dog options : { name : 'Barkee', type : 'Poodle', age : 4 }, // initialize is a MooTools method/constructor that executes the //following function whenever a new instance of a class is created initialize : function( options ) { this.setOptions( options ); }, // Create a method which when passed to a instance of Dog will //tell us our dog is barking. bark : function() { alert( this.options.name + ' is barking.' ); }, // This method is similar to .bark() but will tell us our dog //is sitting. sit : function() { alert( this.options.name + ' is sitting.' ); } });
That's a big code block, but let me break it down for you all. Let's go through what's going on inside the Dog
class.
The Implements
property basically tells our new class what other class properties/methods to include as part of our new class. Either its classes are created by us or our MooTools classes (like Options
and Events)
.
Options
and Events
are MooTools utility classes in the Class.Extras
component of MooTools. Class.Extras
includes the Chain
class (which queues up and executes one function after the next) and Events
class (which adds customizable events to a class). In our case, we're giving our class the instruction to implement the Options
class.
Implements : [ Options ]
The Options
class provides us a way to deal with setting default options for our class, and automatically decides what options to overwrite and leave alone depending on what parameters are passed to it.
It also includes the .setOptions()
method which is the method that triggers the setting of these options.
The options
property lets us set default options for our Dog
class.
options : { name : 'Barkee', type : 'Poodle', age : 4 },
For example, if we don't pass a name
option value when we create an instance of the Dog
class, then it's assumed that our dog's name is "Barkee". Likewise, if we don't pass a value for type, then we're going to assume that it's a "Poodle" (and if your dog is really a Chihuahua, it might get offended, so be sure you set the right type).
The initialize method executes the code block; it's paired with when a new instance of your class is created. In our example, we definitely want to set our class options (name, type, and age), so we write:
initialize : function( options ) { this.setOptions( options ); }
Remember we implemented the Options
class earlier? Well, in doing so, we get access to the .setOptions()
method which does all the hard work of merging our options for us. For example, we don't have to check using if
conditional statements, whether there is or isn't value passed for name or type, and declare what to do in the case that they are or aren't passed—it just does it for us. It sets the options to this
instance.