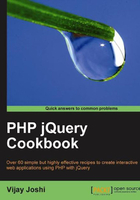
Fetching data from PHP using jQuery
This recipe will teach you the usage of jQuery's get
method to retrieve data from a PHP script. We will see a simple example where data will be fetched from the server using the get
method based on user selection from a form.
Getting ready
Create a directory named chapter2
in your web root. Put the jQuery library file in this directory. Now create another folder inside and name it as Recipe1
. As a recipe can have more than one file, it is better to keep them separate.
How to do it...
- Create a file
index.html
in theRecipe1
folder. Write the HTML code in it that will create a combo box with some options.<html> <head> <title>jQuery.get()</title> <style type="text/css"> ul{border:1px solid black; list-style:none;margin:0pt;padding:0pt;float:left;font-family:Verdana, Arial, Helvetica, sans-serif;font-size:12px;width:300px;} li{padding:10px 5px;border-bottom:1px solid black;} </style> </head> <body> <form> <p> Show list of: <select id="choice"> <option value="">select</option> <option value="good">Good Guys</option> <option value="bad">Bad Guys</option> </select> </p> <p id="result"></p> </form> </body> </html>
- Just before the
body
tag closes, include the jQuery file and write the code that will attach achange
event handler on the combo box. The handler function will get the selected value from the combo box and will send an AJAX request to PHP using theget
method. On successful completion of the request, the response HTML will be inserted into a paragraph present on the page.<script type="text/javascript" src="../jquery.js"></script> <script type="text/javascript"> $(document).ready(function () { $('#choice').change(function() { if($(this).val() != '') { $.get( 'data.php', { what: $(this).val() }, function(data) { $('#result').html(data); }); } }); }); </script>
- Let's code some PHP and respond to the AJAX request. Create a file called
data.php
in the same directory. Write the code that will determine the parameter received from the AJAX request. Depending on that parameter, PHP will create some HTML using an array and will echo that HTML back to the browser.<?php if($_GET['what'] == 'good') { $names = array('Sherlock Holmes', 'John Watson', 'Hercule Poirot', 'Jane Marple'); echo getHTML($names); } else if($_GET['what'] == 'bad') { $names = array('Professor Moriarty', 'Sebastian Moran','Charles Milverton', 'Von Bork', 'Count Sylvius'); echo getHTML($names); } function getHTML($names) { $strResult = '<ul>'; for($i=0; $i<count($names); $i++) { $strResult.= '<li>'.$names[$i].'</li>'; } $strResult.= '</ul>'; return $strResult; } ?>
- Run the
index.html
file in your browser and select a value from the combo box. jQuery will send the AJAX request, which will get the formatted HTML from PHP and will display it in the browser.
How it works...
When a value is selected from the combo box, the corresponding event handler executes. After validating that the selected value is not blank, we send an AJAX request using the $.get()
method of jQuery.
This method sends an HTTP GET request to the PHP script. $.get()
accepts four parameters, which are described below. All parameters except the first one are optional:
- URL: This is the file name on the server where the request will be sent. It can be the name of either a PHP file or an HTML page.
- Data: This parameter defines what data will be sent to the server. It can be either in the form of a query string or a set of key-value pairs.
- Handler function: This handler function is executed when the request is successful, that is, when the server roundtrip is complete.
- Data type: It can be HTML, XML, JSON, or script. If none is provided, jQuery tries to make a guess itself.
Tip
Note that the URL should be from the same domain on which the application is currently running. This is because browsers do not allow cross-domain AJAX requests due to security reasons.
For example, if the page that is sending the request is http://abc.com/, it can send AJAX requests only to files located on http://abc.com/ or on its subdomains. Sending a request to other domains like http://sometothersite.com/
is not allowed.
We specified the URL as data.php
. We sent a key what
and set its value to a selected value of the combo box. Finally the callback function was defined. Since the method is GET, the data that will be sent to the server will be appended to the URL.
Now the request is fired and reaches the PHP file data.php
. Since it is a GET request, PHP's Superglobal array $_GET
will be populated with the received data. Depending on the value of key what
(which can be either good or bad), PHP creates an array $names
that is passed to the getHTML()
function. This function creates an unordered list using the names from the array and returns it to the browser.
Note the use of echo
here. echo
is used to output strings on a page. In this case the page has been called through an AJAX request. Hence, the result is sent back to the function that called it. jQuery receives the response and this is available to us as a parameter of the success
event handler. We insert the received HTML in a <p>
element with the ID result
.
See also
- Sending data to PHP later in this chapter
- Creating an empty page and loading it in parts